In the previous article of Object Oriented programming concepts in PHP, we learned to create a class, define objects for the class and create methods. We also learned about different topics of object-oriented programming like inheritance, interface, and abstraction.
In this article we will deal with php's Magic methods. Php classes are some predefined functions. Magic methods starts with the prefix __, for example __call, __get, __set. There are various magic methods in php. Here we will discuss some of the following magic methods of php which will be use in object oriented programming. First of, let us review some important magic methods with short description:
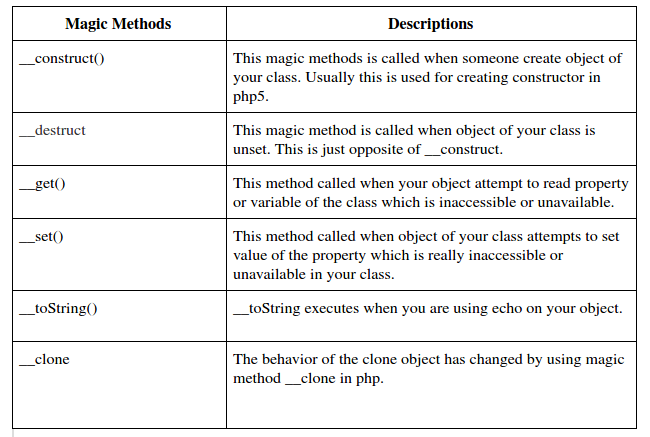
Magic Methods:
The function names __construct(), __destruct(), __call(), __callStatic(), __get(), __set(), __isset(), __unset(), __sleep(), __wakeup(), __toString(),__invoke(), __set_state(), __clone() and __debugInfo() are magical in PHP classes.
We cannot have functions with these names in any of our classes unless we want the magic functionality associated with them.
Now Let us discuss the important Magical Methods,
Constructors:
A constructor is the first function to be called even if n number of functions are there in the class. Constructor need not be initialized and called explicitly. It is automatically called when the object is created with the help of the new operator.
The constructor allows sending the parameter to the class when the object is created.
Examples for the constructor:
Output:
Indian Tiger
An example of constructor in INHERITANCE:
Output:
Calling parent class constructor
Calling parent class constructor
Calling child class constructor
Destructors:
__destruct is the keyword for the destructor. A destructor is called automatically when the object is destroyed. The destructor is automatically called by the garbage collector when the memory allocated for the variable or the object holding the resource is no longer needed.
Example:
Output:
Calling the constructor
Calling Destructor object
__get():
__get() is utilized for reading data from inaccessible properties.
It accepts one argument, which is the name of the property.
It should return a value, which will be treated as the value of the property.
Example:
Note that our student class has $Age commented out, and we attempt to print out the Age value of $obj. When this script is called, $obj is found to not to have an $Age variable, so __get() is called for the Student class, which prints out the name of the property that was requested - it gets passed in as the first parameter to __get(). If you try uncommenting the public $Age; line, you will see __get()is no longer called, as it is only called when the script attempts to read a class variable that does not exist.
__set():
__set() is run when writing data to inaccessible properties.
It accepts two arguments, which are the name of the property and the value.
For Example:
In that script, $mailid is commented out and therefore does not exist in the mytable class. As a result, when $mailid is set on the last line, __set() is called, with the name of the variable being set and the value it is being set to be passed in as parameters one and two respectively. This is used to construct an SQL query in conjunction with the table name passed in through the constructor.
__toString():
The __toString() method is called when code attempts to treat an object like a string.
It accepts no arguments and should return a string.
This allows us to define how the object will be represented.
For Example:
Output:
Hello, how r u
Cloning Objects:
Object cloning is the act of making a copy of an object.When the object is created with the help of new keyword and assigned the object created to a new variable the object reference will be copied but not the value stored in that object. And now if we want to change the value of the object created the copied object variable will also be affected. Similarly, if we change the copied object, the created object data will be affected. So to solve this problem we will use the concept of cloning
For example Copy of the object
Output:
test object ( [a] => no arun [b:test:private] => technology ) test object ( [a] => no arun [b:test:private] => technology ) test object ( [a] => arun [b:test:private] => technology )
Implementation of clone object in PHP:
Object cloning can be implemented by using clone keyword.
Example: Implementation of Object Cloning in PHP
Output:
test object ( [a] => no arun [b:test:private] => technology ) test object ( [a] => no arun [b:test:private] => technology ) test object ( [a] => arun [b:test:private] => technology )
Object cloning with __clone magic method:
Suppose we need to change the value of property in clone object.The behavior of the clone object has changed by using magic method __clone in php.
Example: Object cloning with magic method __clone
Output:
test Object ( [a] => no arun [b:test:private] => technology ) test Object ( [a] => no arun [b:test:private] => technology ) test Object ( [a] => c [b:test:private] => technology }
In this article we explained the important concepts of PHP OOP called Magical Methods.The PHP magic methods are used to react to different events and scenarios that your object might find itself in. Each of these magic methods are triggered automatically, so in essence, you are just defining what should happen under those circumstances. Hopefully each of the examples I’ve presented in this blog will help to you.