This is the fourth post in my series on the AngularJS; check out my initial piece covering ‘An intro to AngularJS’, ‘Data-binding methods’ and ‘Modules & controllers’.
In Angular, Filters are used to format/search functionality in AngualrJS/transform the data. Angular provides default formatter that allows you to display the data in a formatted way to your view or pass the same for the input of another variable. By default, Angular has a list of Filter component in AngularJS and they can be added to your data by including a pipe (|) with expression.
- Uppercase
- Lowercase
- OrderBy
- Number
- Date
- Currency
- Filter
- JSON
- LimitTo
Let’s explore each of them.
Uppercase: Format the data in Uppercase. Sample code:Logged in User Details
- {{n.name | uppercase}}
Uppercase passed in Controller:
We can pass the Filter to Controller by adding $filter argument in controller function. We already have a javascript function to handle uppercase and the same can be achieved in AngularJS as well. Below source code will let you pass the filter in the Controller.
Output:
Hello World
HELLO WORLD
HELLO WORLD
Response: The above source code format the username in uppercase.
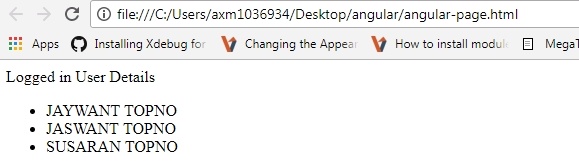
Lowercase: Run the following code to format the data in lowercase.
Sample code:
Logged in User Details
- {{n.name | lowercase}}
Response: The above source code format the username in lowercase. See the screenshot below.
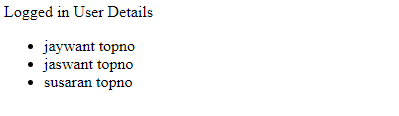
Lowercase passed in Controller:
You can also pass the filter in a controller by adding $filter argument in controller function. Javascript function handles lowercase. Similar to Uppercase, the same can be achieved in AngularJS.
Output:
SAY HELLO TO NEW WORLD ANGULAR!
say hello to new world angular!
say hello to new world angular!
Orderby: Suppose you have a long list of user data: first name, location, job, salary. But when it comes to rendering, how would you like to filter the data in your view? Based on salary/firstname/location. Using orderby, you can achieve this. Have a look at the below code and the result.
Sample code:
- {{ns.name + ', ' + ns.city}}
Response: In the above source code, I have filtered the user data based on the city so the response would look similar to this.
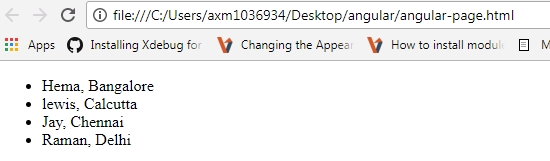
number: It formats data by returning number value in a string.
syntax:
{{ expression | number : fractionSize}}
Here we have expressions that we are formatting the data into a string. With numbers, we have additional parameter fractionize that tells how many digits you can display decimal numbers.
Sample code:
Result:
What if we add price as 707.6758956 and fractionsize: 2
By default, it prints first three digits after decimal point. By adding fractionsize, you can display the number of values of your choice after the decimal.
Will print till next two digits after decimal places.
Input: 77757.677876543
Output: 77757.67
It also prints infinite value when we have something like.
input: 77754323467876432456787654356898765432456787654356787654307.677876543
Output: 7.775e+58
Date: This filter transforms a string date into a human-readable format as listed below:
- yyyy: 4 digit numeric year representation. e.g: 1989, 2017
- MMMM: month name in string format e.g: January, November
- MMM: first three letter of month e.g: JAN, MAR, FEB, DEC
- HH: 24 hours time format e.g: 24, 01,17
- mm: minutes in double-digit format. e.g: 34, 59
Similar to that there are various formatters in AngularJS official documentation. https://docs.angularjs.org/api/ng/filter/date
Syntax:
{{ DateExpression | date : format : timezone}}
Here DateExpression is a timestamp.
The format of an optional parameter. String either would be medium, short, full date, long date etc. by default it takes medium Date.
timezone is also an optional parameter that is used for formatting. e.g: +0530, +0430.
Sample: {{1288323623006 | date:'medium'}}
Result: The above codebase is printing medium date format includes MMM d, y h:mm:ss a
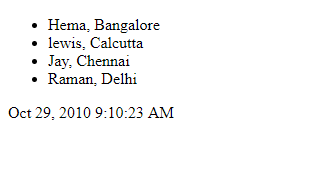
Currency: Add Currency prefix. By default, it takes location currency symbol.
Syntax: {{ expression | symbol : fractionSize}}
Expression is the amount that needs to be manipulated.
Symbol: It is an optional parameter. Placeholder for currency symbol e.g: USD, $, ₹
Fractionsize: It shows a number of digits after the decimal. Add 0 if you do not want to display any value after the decimal.
Sample code: {{20000000000 | currency: "₹" }}
result: ₹20,000,000,000.00
Filter: Technically, Filter is used to return a new array when an item is found. It is also used to get the matching element from the array.
Syntax: {{ filter_expression | filter : expression : comparator : anyPropertyKey}}
filter_expression: source array
expression: element that you are looking for whether string, object, function.
anyPropertyKey: Is an optional parameter. Element needs to be searched.
In the below codebase, we have filtered the output data based on name having ‘i’ and gender ‘m’. Passing multiple filter value treat as & operator. Change the filter value to get the desired result.
Sample code:
Output:
Jani,m
Birgit,m
Demo Dynamic User Filter Codebase:
Json: Converts javascript objects to JSON. Primarily, it is used to debug the applications.
Syntax: {{ expression | json : spacing}}
expression : data to be filtered.
spacing: indentation . default is 2.
Sample code:
The below source code formats the data in JSON format with indentation is set to 8.
Output:
{ "name": "Alfreds Futterkiste", "city": "Berlin", "country": "Germany" }
LimitTo: This filer returns a string or array having a specific number of elements. The counting number of character in the string, array or digits can be taken from either from the beginning or the end.
Syntax: {{ expression | limitTo : limit : begin}}
expression: containing array,string, number etc.
Limit: It returns string length. If it’s in –(negative) then it will start from the end or else from the beginning.
Begin: It is an optional parameter and used to offset from the end of input. The default is 0.
Sample code:
Output:
Try to change the beginning and limit value and see the response.
eraser
sharpener
tape
So far we have gone through almost all the filters provided by AngularJS. Hope you must have used them in real time scenario. In case you have any question or suggestion then please comment below.